TypeScript vs. JavaScript: Differences and Benefits
TypeScript is an open-source language by Microsoft that compiles to JavaScript. In 2019, it was the 7th most-used and 5th fastest-growing language on GitHub. It’s a superset of JavaScript, meaning all valid JavaScript code is also valid TypeScript code. TypeScript adds optional types, providing support for tools for large-scale JavaScript applications for any browser, host, or operating system.
Initially linked with Angular, TypeScript can be used across various frameworks, including React for frontend, Node.js for backend, and React Native for cross-platform apps. We talk a bit more about the relationship between these technologies in our comparison of React and Angular.
How TypeScript Enhances JavaScript
TypeScript doesn’t alter JavaScript; it expands it with valuable features. The TypeScript compiler transpiles TypeScript code into plain JavaScript. Anything that can be written in JavaScript can also be written in TypeScript:
This means you can write React apps in TypeScript without any hassle!
The key enhancement TypeScript brings to JavaScript is static typing through a type system. “Type” defines the intended use of given data (e.g., numeric, Boolean, or string types).
JavaScript is dynamically typed, meaning a variable can contain text, a number, or even a database entity. TypeScript, however, strictly defines what a variable can contain.
For example, if a developer writes an addNumbers function that is defined to only accept two numbers, TypeScript will return an error if another developer tries to input text. In contrast, JavaScript would allow such input without any errors.
Advantages of TypeScript's Static Typing
- Strict Typing: Ensures variables remain consistent.
- Structural Typing: Safeguards data structures.
- Type Annotations: Clearly defines data types.
- Type Inference: Automatically assigns data types.
- Type Checking: Provides compile-time type checking to ensure types match before running the code.
“To me, the main advantage of TypeScript are the autosuggestions. At the moment, I’m coding in JavaScript and this is what I miss the most about TypeScript. Having to jump files back and forth just to see the type gets tiring quickly. My personal TypeScript success story is that thanks to it I have been able to convince our Java-heavy team to write new portions of our application for the web and move away from our legacy Swing/JavaFX stack in favor of React. Developers who are used to statically typed programming languages simply cannot live without the guidance of a compiler. You need something like TypeScript to sway them over to the web or Node.js.”
– Dawid Czarnik, Node.js Developer at STX Next
Additional Features of the TypeScript Compiler
TypeScript isn’t just about types; it supports TypeScript features such as classes, modules, and arrow function syntax, making it ideal for large-scale JavaScript in diverse environments. Microsoft aimed to create a JavaScript-based language that appealed to developers familiar with object-oriented languages like Java or C#.
Benefits of TypeScript
Precise Typing System
TypeScript’s typing system detects errors early during the compile-time, rather than at runtime, reducing the likelihood of bugs reaching production.
Types Make Code Management Easier
Early error detection in development environments like WebStorm improves code management. Types also serve as self-documenting code, clarifying usage without extensive documentation of the source code. TypeScript supports external libraries effectively, providing type declarations directly from the development environment.
Increased Team Performance
Strict typing aids developers in working independently, reducing interruptions and enhancing focus as they write code. Easier onboarding for new developers with explicit data structures and type annotations. TypeScript boosts overall performance and profits by streamlining development processes.
“Based on my experience, TypeScript offers particularly significant benefits in medium-sized and large projects. I’d encourage developers to create these projects in TypeScript from scratch since migrating the existing codebase always takes a lot of work, even when you go for a ‘soft’ strategy.”
– Krzysztof Kęsy, JavaScript Developer at STX Next
Industry Trust and Popularity
Widely adopted by major companies like Microsoft (duh!), Slack, Asana, and many others, TypeScript is often used in popular frameworks and libraries. It's recognized for its effectiveness in large, complex projects and data-driven industries.
Here’s how often TypeScript was downloaded from NPM over the last two years:
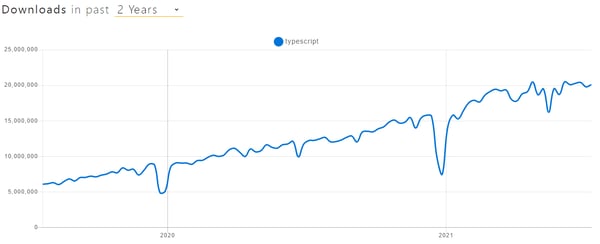
Some numbers from TypeScript’s GitHub repository:

According to StackOverflow’s 2020 Developer Survey, TypeScript is currently the second most loved programming language:

And here are the results of The State of JavaScript – the most popular survey among JavaScript developers:

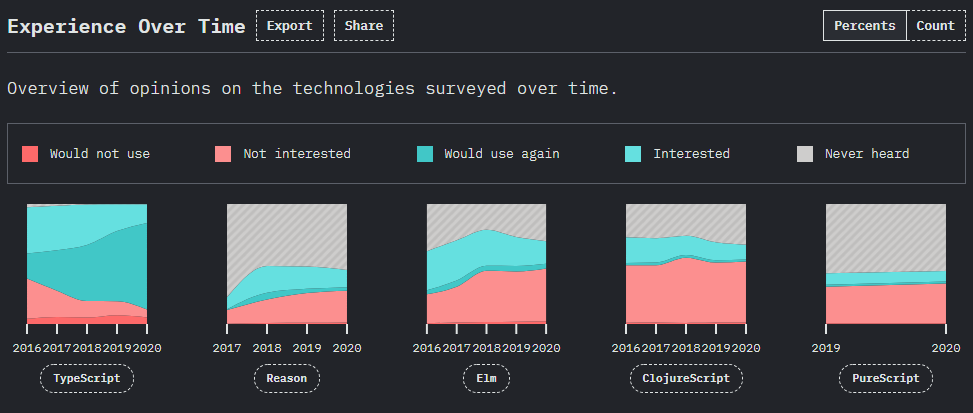
“JavaScript codebase can seem a bit chaotic in its raw form – TypeScript helps to structure it better. It's especially beneficial in larger, complex projects, and a game changer in data-oriented industries such as finance, product data management, etc. Even though it means dealing with lots of rules and limitations, in the end, it makes application development more predictable, thus easier.”
– Jakub Krymarys, JavaScript Developer at STX Next
Disadvantages of TypeScript
- Complex Typing System: It can be complicated to use properly.
- Compilation Required: TypeScript needs compilation, unlike JavaScript, though most modern applications already include a build step.
- False Sense of Security: Over-relying on TypeScript's type checks can lead to complacency, as it only checks types during compilation and not at runtime.
TypeScript advantages and disadvantages: an STX Next’s Developer’s view
We asked our programmers what they really like and dislike about working in TypeScript. Here’s what Przemysław Lewandowski, one of our Senior JavaScript developers, thinks about it.
If you know when to use TypeScript and it’s configured to match your needs, it can be a massive advantage over plain JavaScript. Having types is beneficial if you’re new to a project. You’ll immediately know the exact parameters that each function requires or the complicated structure it returns.
Combined with proper naming and code splitting, typing is another tool for writing readable, self-explanatory code that does not need additional commentary.
When me and my colleagues at STX Next introduced it to a plain JavaScript project in fintech that we took over, it worked well in the new parts of the application. We no longer needed to read through extensive function compositions to know what their outcome would be.
It also helped us to organize and standardize complex financial data structures with a lot of nested objects.
Lastly, it helped us to avoid numerous issues in situations where types would not match. Shortly put, we had more specific, better documented, and more bulletproof code of higher quality.
However, as with everything else, TypeScript has its disadvantages. Setting TypeScript too strictly, for example, having to manually type everything without relying on type inference, can be tiring and frustrating.
Also, if you need to prototype something quickly, I would personally pick plain JavaScript as it gives me greater freedom and less code to write.
You also need to be careful about introducing new libraries to a TypeScript project—adding type definitions to them is still not a standard. That one library that matches your needs does not have to have them written. You will either have to write them yourself or look for another one.
What I also found a bit more demanding with TypeScript is writing unit tests. When you are just learning TypeScript, mocking things is challenging, as everything demands getting specific types with all their properties. In JavaScript, you would not have to worry about that. Just pass the object with only the properties that you need in this particular test.
Alternatives to TypeScript
Several languages offer strict type systems and compile to frontend code, but TypeScript’s familiarity makes it easier for JavaScript developers to adopt.
- TypeScript vs. Flow: Flow is popular but lacks TypeScript's broader feature set and support.
- TypeScript vs. JSDoc: JSDoc supports type annotations but is less seamless than TypeScript’s integrated typing.
Migrating to TypeScript with Visual Studio Code
Having read all the praise for TypeScript, you might be wondering how your JavaScript projects can benefit from it. Well, there’s a good chance they already are! Advanced development environments like WebStorm use TypeScript type definitions to facilitate code development with external libraries. Additionally, editors like Visual Studio Code offer built-in TypeScript support, displaying errors as you write and supports TypeScript in binding expressions within Vue templates and integrating TypeScript with ESLint for linting TypeScript code.
That said, this is only a partial benefit. TypeScript compiles to readable, standards-based JavaScript, ensuring your code adheres to industry standards. So, can ongoing projects move easily to TypeScript?
The answer is: yes, they can. And the transition likely won’t be as time-consuming as it may seem. Here are two ways to perform such a migration:
The Hard Way: Everything in TypeScript at Once
Simply rewriting all your code to TypeScript is the most obvious but also the most challenging and time-consuming method. Valid JavaScript code is also valid TypeScript code, so you mostly change file extensions, run the compiler, and fix typing errors. This is best suited for small projects and teams, as it requires halting development to complete the migration. The advantage is it's future-proof, erasing concerns about legacy JavaScript code.
The Soft Way: Only New Things in TypeScript
Thanks to TypeScript's interoperability with JavaScript, you can write TypeScript code alongside JavaScript code. This means you don't need to move your whole project at once. Spend a few hours configuring TypeScript, and then ensure each new feature is written in TypeScript.
Gradually, old code can be updated to TypeScript as it’s edited. This approach works best for medium- and large-sized projects, allowing your team to benefit from TypeScript immediately without halting development. The downside is dealing with legacy JavaScript code later on, and some segments of your TypeScript code may be poorly typed where old code was used.
Despite those small drawbacks, I'd still recommend choosing this way if you decide to include TypeScript in your ongoing project.
Final thoughts on TypeScript
TypeScript enhances JavaScript development, supports industry giants, and simplifies managing large projects. By offering strict typing and additional features, TypeScript makes code more reliable and easier to understand, leading to fewer bugs and faster releases.
Will your project be the next in line to join the frontend revolution? It’s up to you to decide!
Thank you for reading our article on the benefits of using TypeScript for your frontend development. We hope you’re going to give this awesome solution a shot in your next software project.
Do you have any questions or comments about TypeScript or software development in general? Feel free to leave them here, and we’ll happily get back to you.
And if you need frontend or backend support of any kind, our door is always open!